Master JavaScript Like A Champ.
Top 20 JavaScript Interview Questions and Answers in 2023.
Q1. What are first-class functions?
In some programming languages, there is a concept of first-class functions. First-class functions are those treated like ordinary variables.
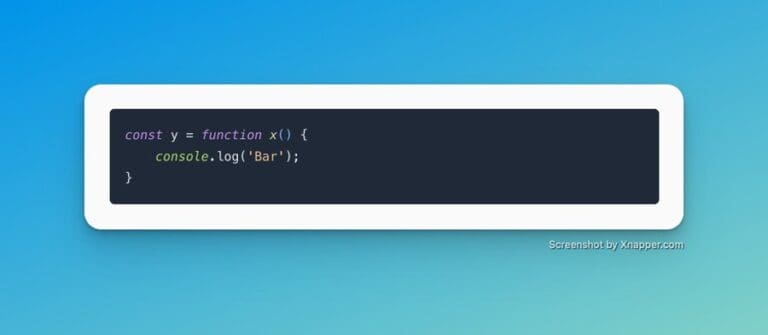
Q2. What are the function statements and function expressions?
When a function gets created using the `function` keyword, it’s called a function statement. Assigning a function to a variable is a function expression.
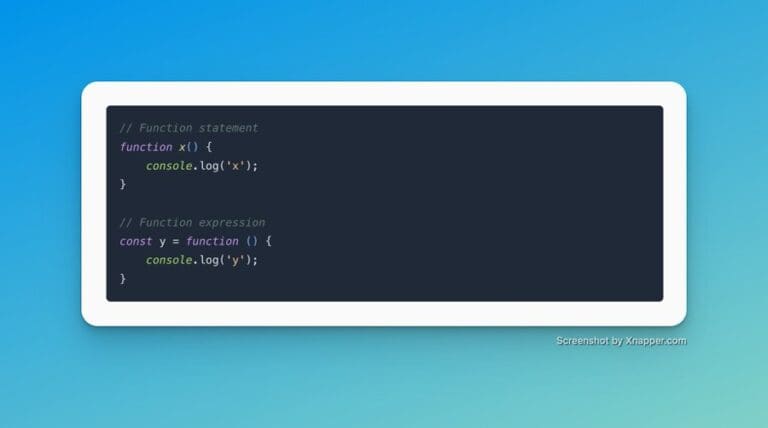
Q3. What are first-order functions?
A first-order function does not take another function as an argument or return a function.
Q4. What are higher-order functions?
Higher-order functions can accept a function as an argument or return a function as a result.
Q5. What are pure functions?
Pure functions are those whose return values only depend on the arguments they receive. We get the same return value if we call a pure function with the same arguments.
Q6. What is function currying?
Function currying is a process in which we convert a function with multiple parameters to a chain of functions with a single parameter.
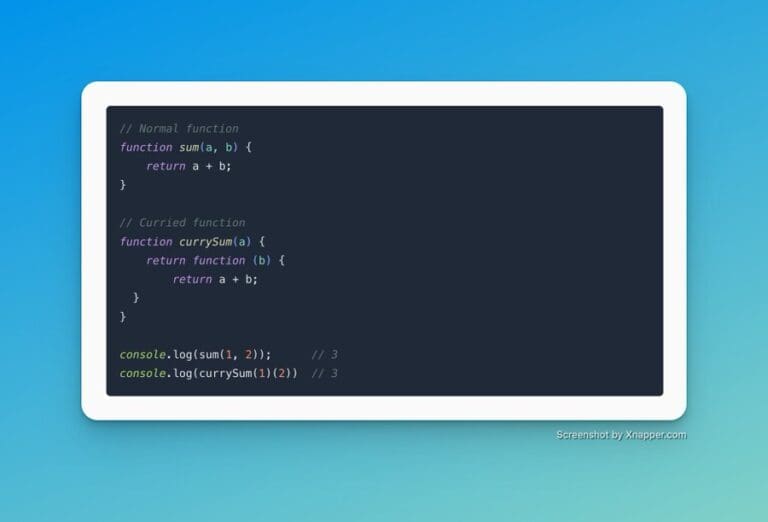
Q7. What is prototype chaining?
Prototype chaining is the process of building new objects from old ones. Essentially, it’s like inheritance in a class-based language.
Q8. What is the difference between the null and the undefined values?
Variables get assigned a `null` value to indicate they are declared and assigned but don’t point to anything. An `undefined` variable is declared but not initialized.
Q9. What is a strict mode in javascript?
ECMAScript 5 introduced a strict mode that executed the programs and functions strictly. To use strict mode, we write “use strict”; on the top of our JavaScript file.
Q10. What is the difference between == and ===?
The `==` operator is also called a type-converting equality check operator. When we compare values of different data types, it attempts to do type conversions before checking the equality.
The `===` operator is also called a strict equality operator. JavaScript engine will make no type conversions in this case.
Q11. What are arrow functions?
An arrow function is a compact way of declaring a function. Functions declared using arrow syntax don’t have this, arguments bindings.
First-class cannot use these functions to create a class constructor.
Q12. What is JSON?
JSON stands for JavaScript Object Notation. It stores data in a format similar to JavaScript objects.
Q13. What is hoisting?
Hoisting is the process by which the JavaScript engine sets aside memory for variables, functions, and classes to give the impression that they are at the top of the code.
Q14. What is memorization?
Memorization is a technique that saves calculated results to improve a function’s efficiency.
The arguments given to the function serve as the cache object’s key. If a key is present, the function returns the result immediately.
Otherwise, the function computes the result, saves it in the cache, and returns it.
Q15. What are closures?
A function bound to its immediate surroundings creates a closure.
Essentially, a function defined inside another function is a closure.
The variables and operations of the outer function are accessible to the inner function.
Q16. What is scope in JavaScript?
A scope is a code section allowing us to access specific variables and functions.
It determines the visibility of variables and functions.
Variables and functions declared within a particular scope cannot be accessible outside the scope.
Q17. What is the difference between local storage and session storage?
Both local storage and session storage store data.Â
Local storage persists the data even when we close the browser.
In contrast, session storage removes the data when we close the browser tab.
Q18. What is a JavaScript promise?
A promise is a JavaScript object that may or may not produce a value in the future. In other words, the promise object yields a single value when resolved.
A promise object can have one of three states: pending, fulfilled, and rejected.
Q19. What is a callback function?
A function passed as an argument to some other function is a callback function.
We will invoke the callback function within the function to accomplish the desired result.
Q20. What is callback hell?
Callback Hell is a pattern that consists of multiple nested callback functions.
It is an anti-pattern because the code is hard to read and modify.